Running an Action from Alli Marketplace
There is a special endpoint on actions specifically for kicking of actions runs from forklift: /forklift/webhook/{actionId}
where {actionId}
is the value provided when setting the datasource in Alli Actions.
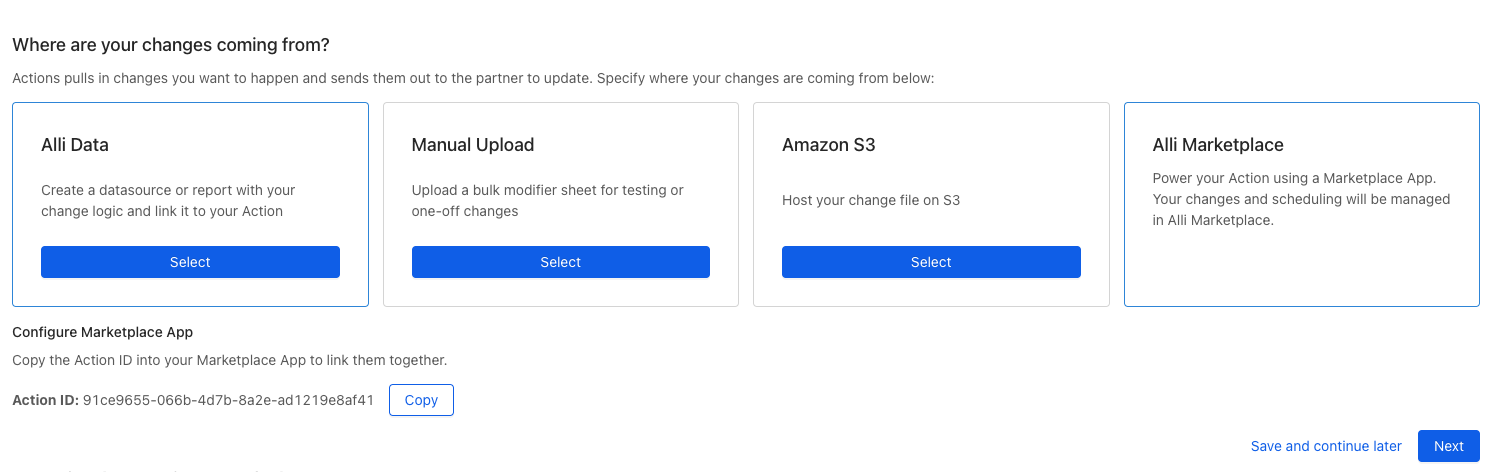
Overriding Data Sources
Actions being kicked off from Marketplace must override the Action’s Data Source so the action can run with real data. Set the datasource
key in the request body to do this.
S3 Data Sources
Actions can read data from S3 and will read from the actionsprod-imports
bucket by default if no information other than files
is supplied:
request_body = {
'datasource': {
'type': 's3',
'configuration': {
'files': ['some/file/on/s3.csv'],
}
}
}
If you’d like to use a custom S3 bucket, you must specify bucket
, access_key_id
, and secret_access_key
(and, optionally, region
which will defaul to us-east-1
).
request_body = {
'datasource': {
'type': 's3',
'configuration': {
'files': ['some/file/on/s3.csv'],
'bucket': 'some-custom-bucket',
'access_key_id': 'AKAIxxxxxxx',
'secret_access_key': '******',
'region': 'us-west-2',
}
}
}
Alli Client File Data Sources
Using Alli’s Client Files (see How to Read and Write Files to a Client's S3 Bucket ) is a great way to share data between Alli Marketplace and Alli Actions without the need for credential sharing. Write your file to Alli’s Client S3 Bucket and then send the filename as part of the request body. Use the datasource type alli+files
:
request_body = {
'datasource': {
'type': 'alli+files',
'configuration': {
'files': ['some/file.csv'],
}
}
}
If you’d like to use pattern matching to grab a set of files, you may use glob://path/to/file_*.csv
where the *
matches zero or more characters.
request_body = {
'datasource': {
'type': 'alli+files',
'configuration': {
'files': ['glob://some/file*.csv'],
}
}
}
This will match all files in the some
directory that are prefixed with file
and end with .csv
. For example: some/file_1.csv
and some/file_2.csv
.
Alli Data Data Sources
Alli Actions cannot read Alli Data tables outside of the client’s namespace. So an action running for Acme could not read data from a table for Wayne Enterprises.
Alli Actions leverage’s Alli’s Client Credentials system to security connect to Alli Data and read tables or views into actions. You’ll use a datawarehouse
datasource type to do this:
request_body = {
'datasource': {
'type': 'datawarehouse',
'configuration': {
'table_or_view': 'client_slug_here.table_or_view_here',
}
}
}
Other Request Body Parameters
In addition to datasource
described above, you may send pallet_id
and pallet_name
. These values are shown to the user in Alli Actions as part of the trigger that kicked off the action.
request_body = {
'pallet_id': os.environ.get('FORKLIFT_PALLET_ID'),
'pallet_name': os.environ.get('FORKLIFT_PALLET_NAME'),
'datasource': {
# see above
}
}
Authentication
Any requests made to a /forklift/*
endpoints require HTTP Basic Authentication with the username forklift and a password that can be found in the Actions 1password vault named Forklift API Credentials.
Here's an example in Python with requests.
import os
import pprint
import requests
from requests.auth import HTTPBasicAuth
resp = requests.post(
'https://actions.pmg.com/forklift/webhook/90720784-8917-4671-bd05-f67a8f198c8e',
json=request_body, # see above for what `request_body` looks like
auth=HTTPBasicAuth('forklift', os.environ['ACTIONS_PASSWORD']) # your marketplace app or template should set `ACTIONS_PASSWORD` in its environment variables
)
pprint.pprint(resp.json())